Jest: A JavaScript Testing Framework
Unit testing is an essential practice in any software development project. It involves testing individual components or units of code to ensure they work as intended. With the rise of new frameworks and technologies, it's important to incorporate unit testing into your development workflow.
Jest: An Overview
Jest, the JavaScript testing framework created by Facebook, stands as a testament to originality and innovation. It's an open source tool that helps developers write automated tests for their JavaScript code. Jest provides a simple and powerful way to test your code and ensure that it works as expected. It's also very easy to set up and use, making it a popular choice for many developers.
Features of Jest
Jest has several features that make it a powerful testing tool for JavaScript developers. A few features are below:
Easy to use: Jest is easy to set up and use, even for developers who are new to testing. It has a simple and intuitive API that makes it easy to write tests for your JavaScript code.
Fast and efficient: Jest runs tests in parallel, making it faster and more efficient than many other testing frameworks.
Snapshot testing: Jest allows you to take snapshots of your code and compare them to previous snapshots to ensure that nothing has changed unexpectedly.
Mocking: Jest has built-in mocking support, allowing you to simulate different scenarios in your tests.
Code coverage: Jest can generate code coverage reports to show you how much of your code is covered by your tests.
Integration with other tools: Jest integrates with other tools like Babel, webpack, and ESLint, making it easy to incorporate into your development workflow.
Using Jest in a Node.js based Project
To incorporate Jest into a Node-based project, follow these steps for creating and installing Jest within the project:
1. Begin by creating a folder with the desired project name, such as "jestNodeProject.
2. "Use the terminal or command line to navigate to the project directory and execute the npm init script with the following command:
3. Upon executing the above command, you will be prompted with various questions and parameters for project details, including the project name, version, etc. Simply press enter to accept the default values. After completion, a package.json file will be created in the project directory. This file is crucial for configuring any node-based project.
4. Install the Jest package into the newly created project by executing the following command:
This command will install the Jest module and its dependencies.
5. At this point, the node project is ready with Jest bindings. Update the package.json file , for configure the npm test script and run the Jest tests, by adding the following script section:
The final package.json file will resemble the example shown below:
You have successfully integrated Jest into your Node-based project and configured the npm test script to execute Jest framework-based tests by completing these steps.
Writing some sample tests for a JavaScript function
When it comes to writing tests for a JavaScript function, we'll start by creating a code snippet that performs addition, subtraction, and multiplication of two numbers. Subsequently, we'll develop the corresponding Jest-based tests. Let's examine the code for our application or function under test.
Within the node project established in the previous section, create a JavaScript file named "calculator.js" with the following content:
Next, generate a test file named "calculator.test.js" within the same folder. Jest expects this naming convention to identify files containing Jest-based tests. Also, import the function under test to execute the code within the test. Here is the initial file with the import/require declaration:
Now, let's proceed to write tests for the various methods in the main file (sum, diff, and product). Jest tests follow a behavior-driven development (BDD) style, utilizing a primary "describe" block for each test suite, which can contain multiple "test" blocks. It is worth noting that tests can have nested "describe" blocks as well.
For example, let's write a test that verifies the addition of two numbers and validates the expected result. We will provide the numbers 1 and 2, expecting the output to be 3.
Regarding the test above, please consider the following points:
a) The "describe" block serves as an outer description for the test suite, acting as a container for all the tests written for the calculator in this file.
b) Next, we've an individual "test" block, representing a single test case.The string inside quotes denotes the test name.
c) Observe the code within the "expect" block. "expect" functions as an assertion. The statement calls the "sum" method from the function under test, passing in inputs 1 and 2, and expects the output to be 3.
To enhance readability, we can rewrite the code in a simplified manner:
To run this test, simply execute the command "npm test" in the terminal or command prompt at the project location. The output ought to seem as follows:
By following this approach, you can write effective tests for JavaScript functions using Jest, ensuring the accuracy and reliability of your code.
Here we are expecting a sum of 1 and 2 to return 10 which is incorrect.
Let’s try executing this and see what we get.
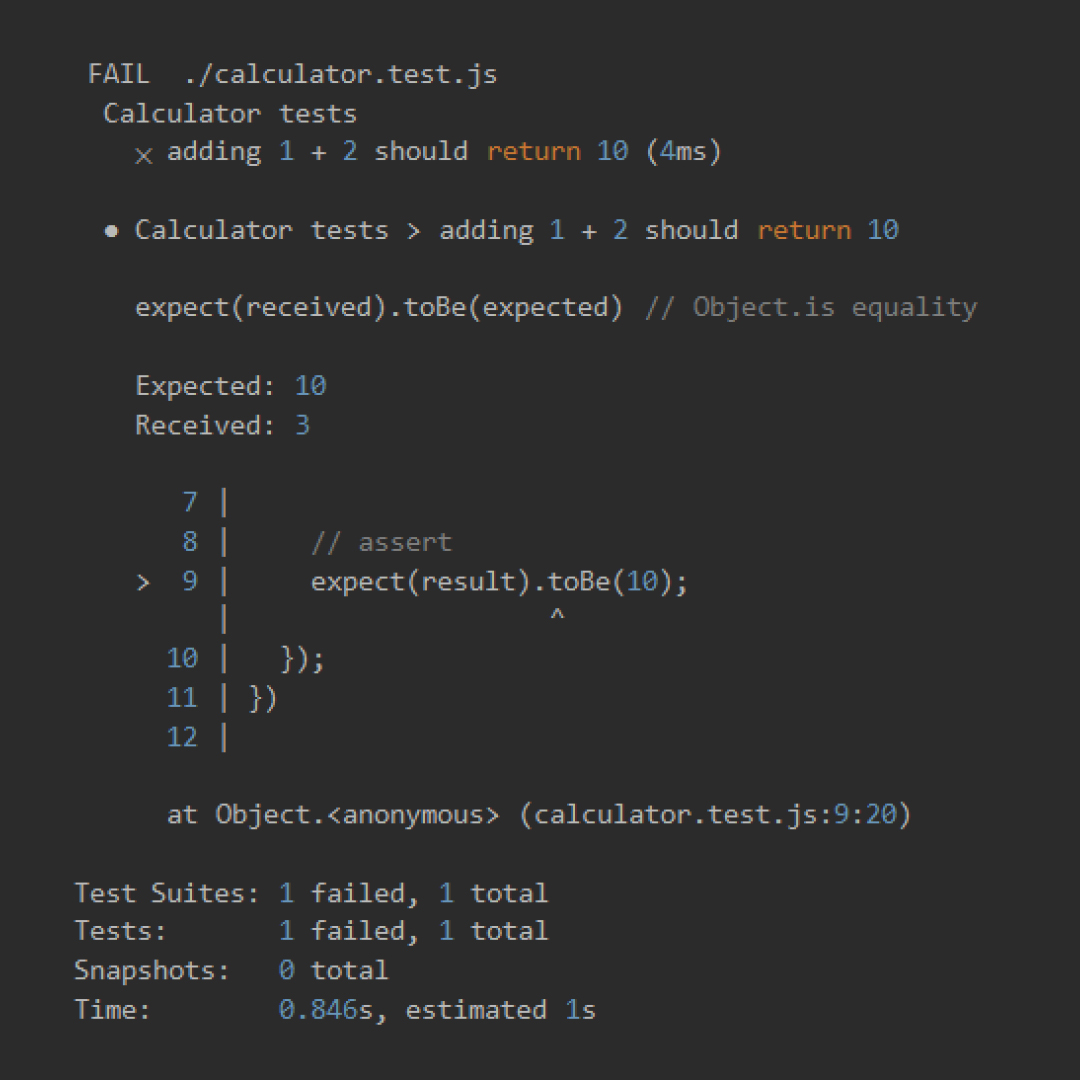
You can see that detailed output when a test is failed i.e. what was actually returned and what was expected and which line caused the error in the function under test etc.
b) Let’s write more tests for the other functions i.e difference and product.
The test file with all the tests will look as shown below.
When the above tests are executed, the output given below gets generated.
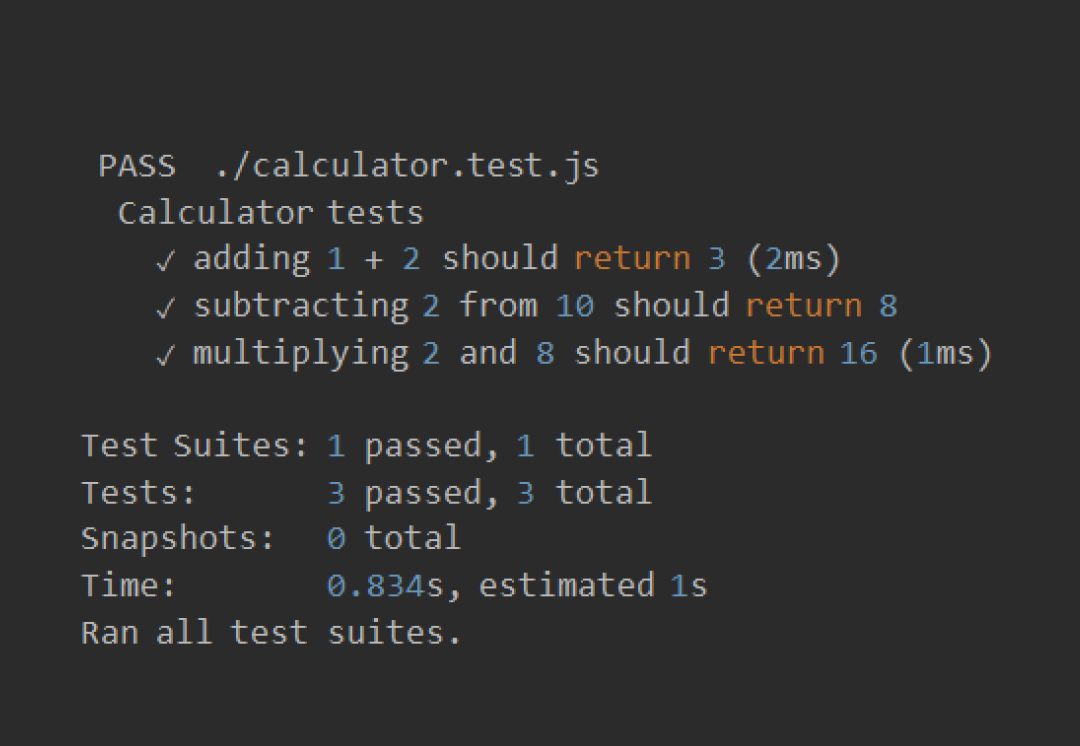
Jest Hooks - Initialization and Cleanup
Similar to other xUnit-based unit test frameworks, Jest provides hooks for initialization and cleanup methods. These hooks execute before and after each test within the test suite or before and after the execution of the entire test suite. A total of four hooks are available for use:
- beforeEach and afterEach: These hooks run before and after each test in the test suite.
- beforeAll and afterAll: These hooks run only once for each test suite. For example, if a test suite consists of 10 tests, these hooks will execute only once for the entire test suite.
Now, let's incorporate these hooks into the existing test example for adding two numbers. We will set the inputs in the beforeEach hook for illustration purposes. The test file would include the test hooks as shown below:
Conclusion
Performing unit testing is an essential component of software development projects, and it holds particular significance when engaging with a novel framework. Jest, an influential and user-friendly testing framework designed for JavaScript developers, offers a wide range of capabilities that make it an excellent option for testing JavaScript code. Notable features encompass snapshot testing, mocking, and code coverage. If you haven't yet incorporated Jest into your development workflow, it is certainly worthwhile to explore its capabilities!