Modern Design Practices: Jetpack Compose
What is Jetpack Compose?
Well, what does that mean?
In Android, we normally create XML files for layouts and then update the UI elements every time based on the processing flow or inputs received using Data Binding/View Binding/Kotlin Semantics/findViewById.
But now it’s different!
Compose helps us to write a function that has both UI elements and rules for updating those elements, and these composable functions are called whenever information gets updated, i.e., a part of the UI is recreated every time without performance issues.
Most of the modern frontend toolkits and frameworks such as React.js and Flutter follow this pattern.
Read more: Flutter vs React Native – War of Tech Giants
Why Jetpack Compose?
- Uses fewer lines of code, so easily maintainable
- Declarative Approach – Just describe the UI, compose will do the rest to update our UI with respect to application state changes.
- No more XML, just one language Kotlin
- These features accelerate development time.
Getting started with Jetpack Compose
While composing UI with Jetpack Compose, we need to leave behind various types of layouts and remember just one thing: Everything is a composition of rows and columns. Think of rows and columns as just LinearLayout with orientation as horizontal or vertical respectively.
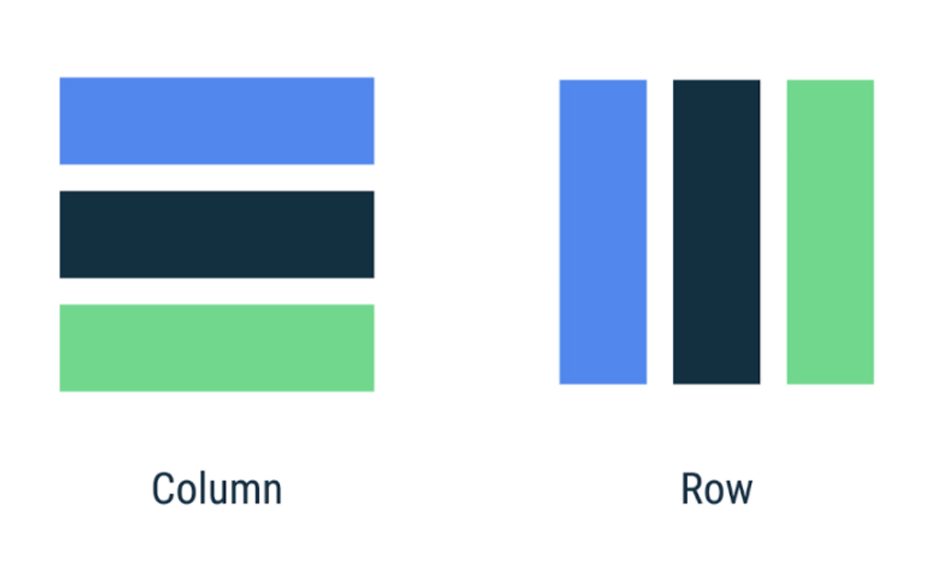
For our first learning experience, let's recreate a Counter App using Jetpack Compose.
For a great Jetpack Compose development experience, you should download Android Studio Arctic Fox.
The latest version of Android Studio features a new project template namely, Empty Compose Activity to help us get started.
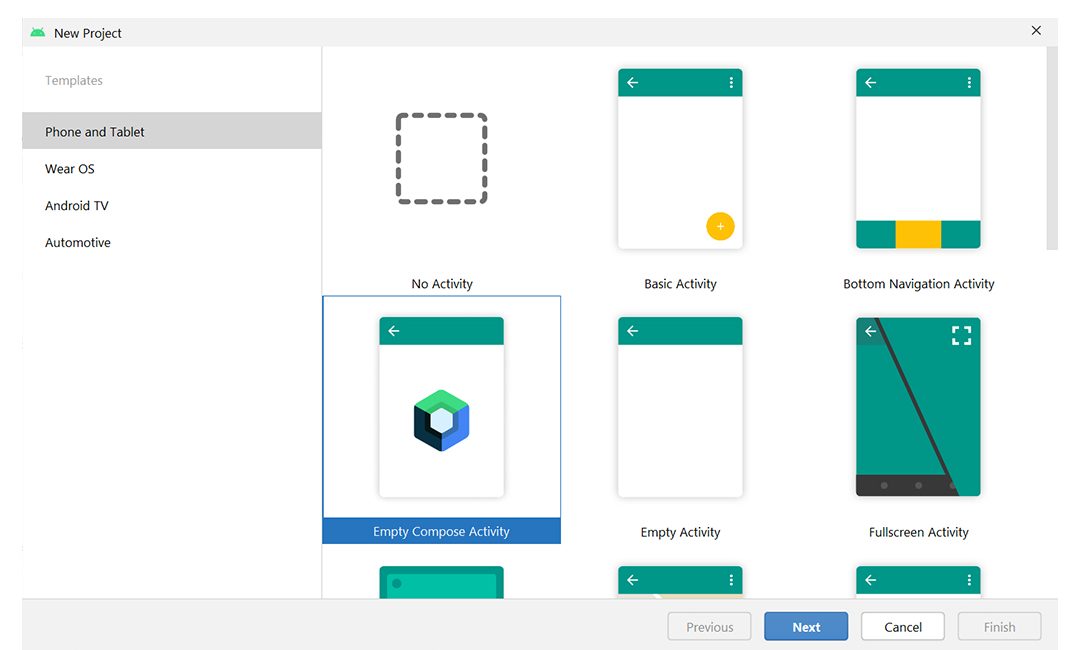
Let’s create a new project with ‘Empty Compose Activity’ template and open MainActivity.kt
Here you might have noticed few changes:
- setContent{} instead of setContentView{} - The setContent{} block defines the activity's layout where we call composable functions.
- @Composable and @Preview decorators - to add a view to the UI, we need to create a function with @Composable annotation, which makes it a Compose function. Compose UI library defines a whole lot of composable functions to display various elements on the screen. For example, the Text() function defined by the Compose UI library displays a text label on the screen.
To preview the UI rendered in Android Studio, we can use @Preview annotation.
- Changes in folder structure – No more XML files.
Lets’ clear all those default code lines and start building an app from scratch.
We’ll create a simple UI with just 3 elements – Appbar, TextView and a Button
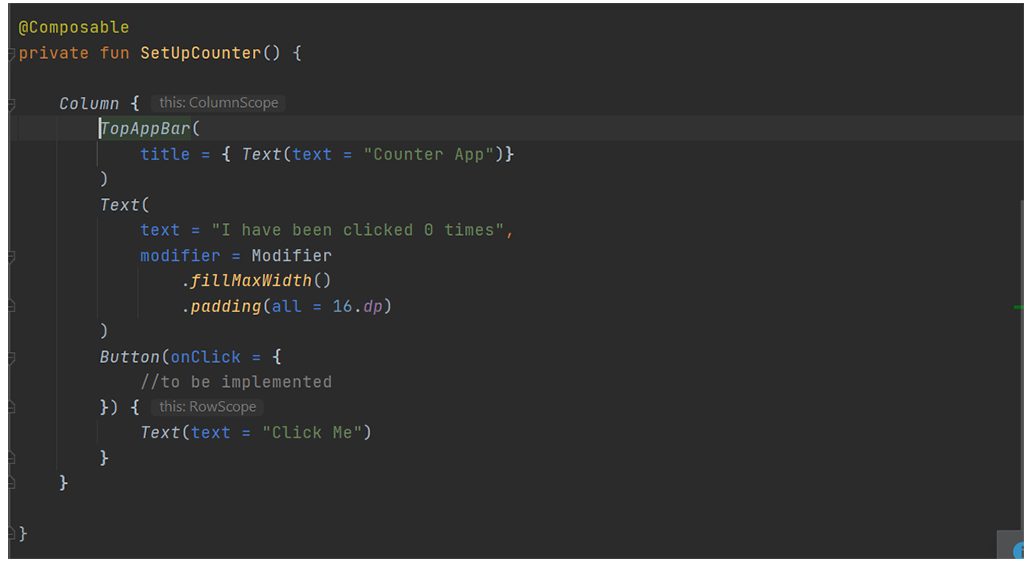
As discussed earlier, we have only rows and columns in Compose UI.
So here, we have created a composable function SetUpCounter, which renders a column of UI elements.
TopAppBar is an inbuilt composable function for adding a top bar to our application. Similar is the case of Button and Text.
You will notice the modifier argument in the Text function. This is the Compose way of adding formatting to the elements, which is uniform across all the elements.
Here we set the Text View’s width to match_parent using fillMaxWidth()
In Button, we have added the onClick() where we will update the counter value and this updated value will be shown in our Text Composable.
So, our onCreate() would look like:
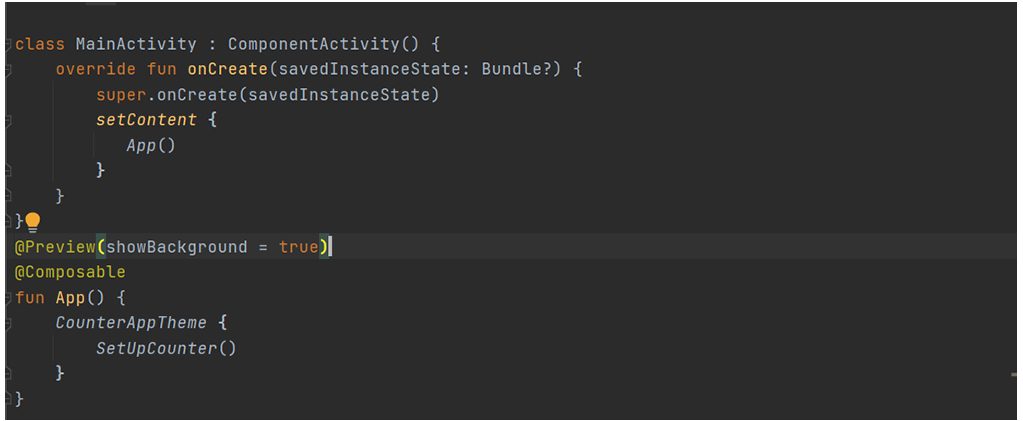
Here, setContent{} calls a composable, preview able function App(), which in turn calls the composable function SetUpCounter() we discussed earlier.
Now our screen looks like this:
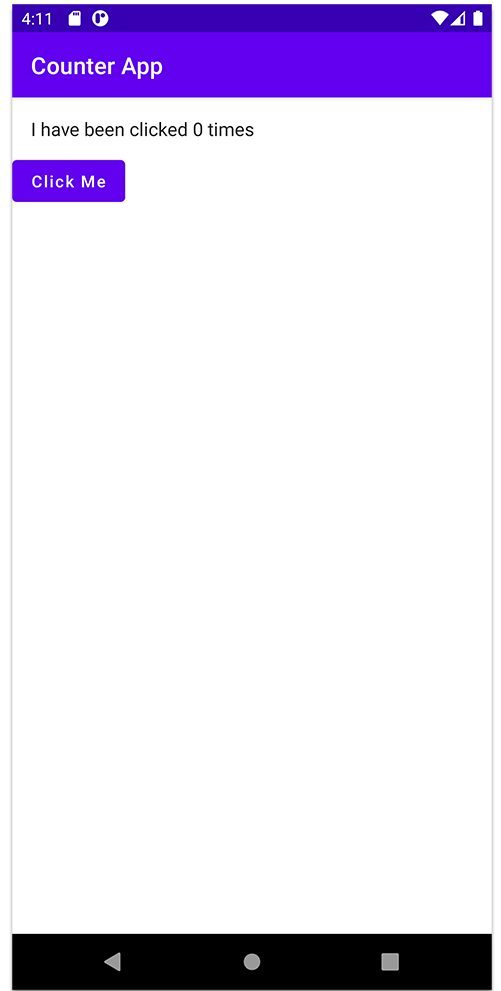
Moving on, let’s implement the counter functionality using states in Jetpack Compose.
What is a State in Jetpack Compose?
State is an object which contains certain data that is mapped to one or many widgets. The value of the state can change during the runtime, and using this value from the state object, we can update the data shown in widgets.
Let’s modify SetUpCounter() to create a variable counterState of type MutableState which will hold an initial value of 0. When the button is clicked, counterState value is incremented by 1 and the state would re-compose to redraw the UI.
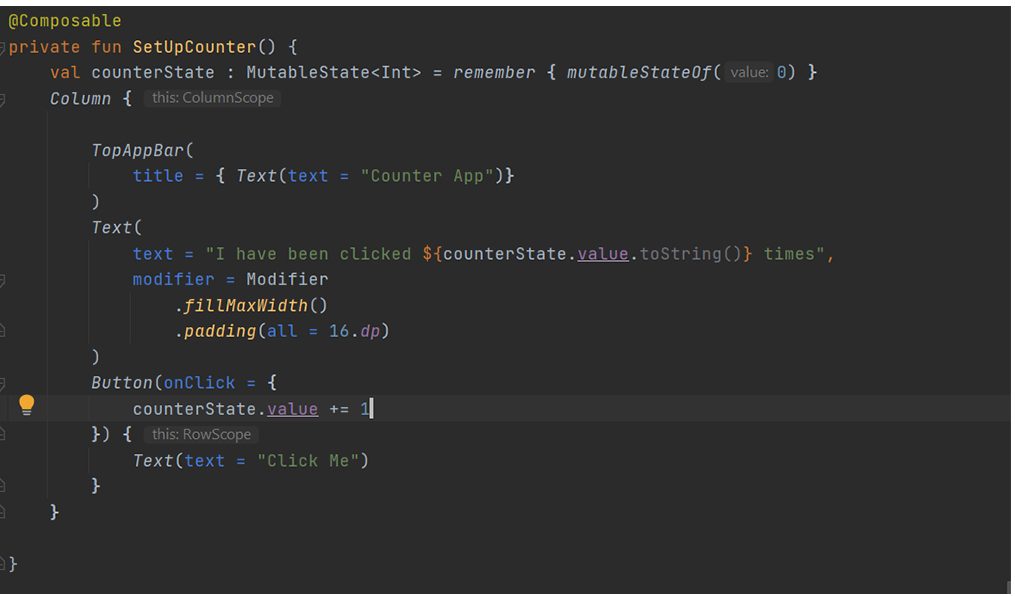
remember is a composable function to cache expensive operations.
Compose observes any reads and writes to the MutableState object and triggers a recomposition to update the UI, thus we get our required output.
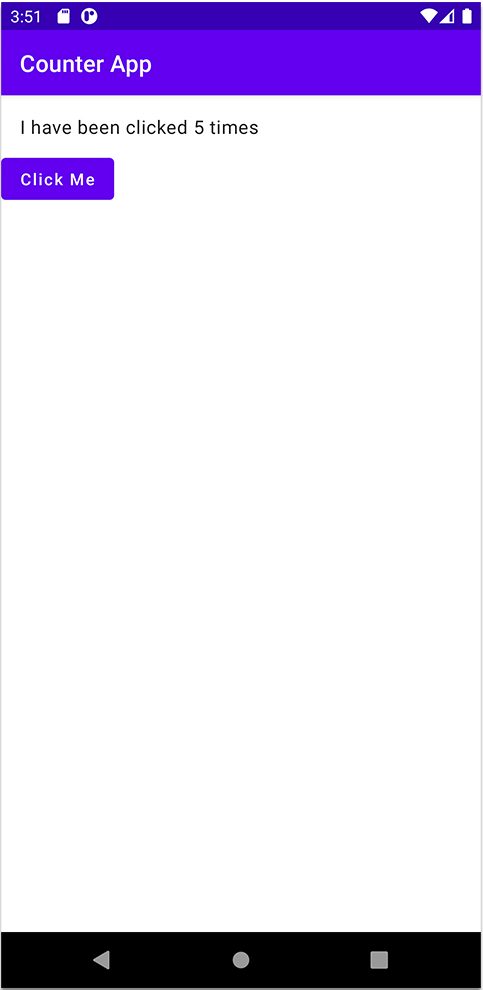
This is just a small introduction to the basics of Jetpack Compose. To explore more please visit the official Android Jetpack Compose Documentation.